共计 3959 个字符,预计需要花费 10 分钟才能阅读完成。
提醒:本文最后更新于2017-10-31 12:12,文中所关联的信息可能已发生改变,请知悉!
对于富客户端的 Web 应用页面,自动登录、页面修改、抓取页面内容、屏幕截图、页面功能测试…面对这些需求,使用后端语言需要花费不少的精力才能实现。此时 SlimerJS、phantomJS 或 CasperJS 或许是更好的一种选择。
一、PhantomJS 和 SlimerJS
PhantomJS 和 SlimerJS 都是服务器端的 JavaScript API 工具,可以理解为无界面的可编程操作的浏览器。 它们大部分的 API 接口都很相似,使用方法也很接近,最大的不同在于:PhantomJS 基于 Webkit 内核,不支持 Flash 的播放;SlimerJS 基于火狐的 Gecko 内核,支持 Flash播放,并且执行过程会有页面展示。
借助 PhantomJS 或 SlimerJS 所提供的 API,你几乎可以使用 javascript 模拟在浏览器上的任何操作:打开页面、前进/后退、页面点击、鼠标滚动、DOM 处理、CSS 选择器、Canvas 画布、SVG画图,如此等等。
例如,对页面的某个区域截图:
SlimerJS 示例:
var webpage = require('webpage').create(); | |
webpage.open('http://www.meizu.com') // 打开一个网页 | |
.then(function() { // 页面加载完成后执行 | |
//保存页面截屏 | |
webpage.viewportSize = { | |
width: 650, | |
height: 320 | |
}; | |
webpage.render('page.png', { | |
onlyViewport: true | |
}); | |
//再打开一个网页 | |
return webpage.open('http://bbs.meizu.com'); | |
}) | |
.then(function() { | |
// 点击某个位置 | |
webpage.sendEvent("click", 5, 5, 'left', 0); | |
slimer.exit(); //退出 | |
}); |
将以上代码保存为 test_slimerjs.js
,然后执行:
slimerjs test_slimerjs.js
PhantomJS 示例:
var webpage = require('webpage').create(); | |
var url = 'http://www.phantomjs.org/'; | |
webpage.open('http://www.meizu.com', function (status) { | |
//打开一个页面 | |
}).then(function(){ | |
//保存页面截屏 | |
webpage.viewportSize = { | |
width: 650, | |
height: 320 | |
}; | |
webpage.render('page.png', { | |
onlyViewport: true | |
}); | |
//再打开一个网页 | |
return webpage.open('http://bbs.meizu.com'); | |
}).then(function(){ | |
webpage.sendEvent("click", 5, 5, 'left', 0); | |
phantom.exit(); | |
}); |
将以上代码保存为 test_phantomjs.js
,然后执行:
phantomjs test_phantomjs.js
可以看到,上面的代码内容非常相似,实现的功能都是打开页面,然后截图。
参考:
http://phantomjs.org/
https://github.com/ariya/phantomjs
http://slimerjs.org/
http://docs.slimerjs.org/current/
https://github.com/laurentj/slimerjs/
二、前端自动化测试工具 CasperJS
CasperJS 是一个开源的导航脚本和测试工具。它提供了一套用于 Web 应用测试的方法组件,这些组件基于 PhantomJS 或 SlimerJS 所提供的 javascript API,实现对 Web 应用的功能执行。CasperJS 简化了完整的导航场景的过程定义,提供了用于完成常见任务的实用的高级函数、方法和语法。如:
- 定义和整理导航步骤
- 表单填充
- 点击、跟踪链接
- 区域、页面截图
- 断言远程DOM
- 日志、事件
- 资源下载,包括二进制资源
- 捕捉错误,并做出相应的响应
- ……
使用 CasperJS 的方法组件,可以更方便的书写前端自动化测试脚本。
CasperJS 示例:
var utils = require('utils'); | |
var webpage = require('casper').create({ | |
//verbose: true, | |
logLevel: 'debug', | |
viewportSize: { | |
width: 1024, | |
height: 768 | |
}, | |
pageSettings: { | |
loadImages: true, | |
loadPlugins: true, | |
XSSAuditingEnabled: true | |
} | |
}); | |
//打开页面 | |
webpage.start() | |
.thenOpen('http://www.meizu.com', function openMeizu(res) { | |
this.echo('打印页面信息'); | |
res.body = '';//不打印body信息 | |
utils.dump(res); | |
//点击登录按钮 | |
if (this.exists("#_unlogin")) { | |
this.echo('点击登录按钮'); | |
this.click("#_unlogin a:nth-child(1)"); | |
this.wait(3000, function wait3s_1() { | |
if (this.exists("form#mainForm")) { | |
this.echo("需要登陆,填充账号信息。。。"); | |
//填充表单账号 | |
this.fill('form#mainForm', { | |
'account': 'lzwy***@flyme.cn', | |
'password': '********' | |
}, true); | |
this.capture('meizu_login_page.png'); | |
this.wait(3000, function wait3s_2() { | |
//登录按钮存在,点击 | |
if (this.exists("#login")) { | |
this.echo('提交登录'); | |
this.click("#login"); | |
} | |
}); | |
} | |
}); | |
} | |
}) | |
.then(function capture() { | |
if (this.exists('#mzCustName')) { | |
this.echo('登录成功!开始截图存储..'); | |
} else { | |
this.echo('登录失败!请查看截图文件') | |
} | |
//截图 | |
this.capture('meizu.png'); | |
this.captureSelector('meizu_header.png', 'div.meizu-header'); | |
}) | |
.then(function exit() { | |
this.echo('执行完成,退出'); | |
this.exit(); | |
}) | |
.run(); |
将以上代码保存为 test_casperjs.js
,然后执行:
casperjs test_casperjs.js
效果参考:
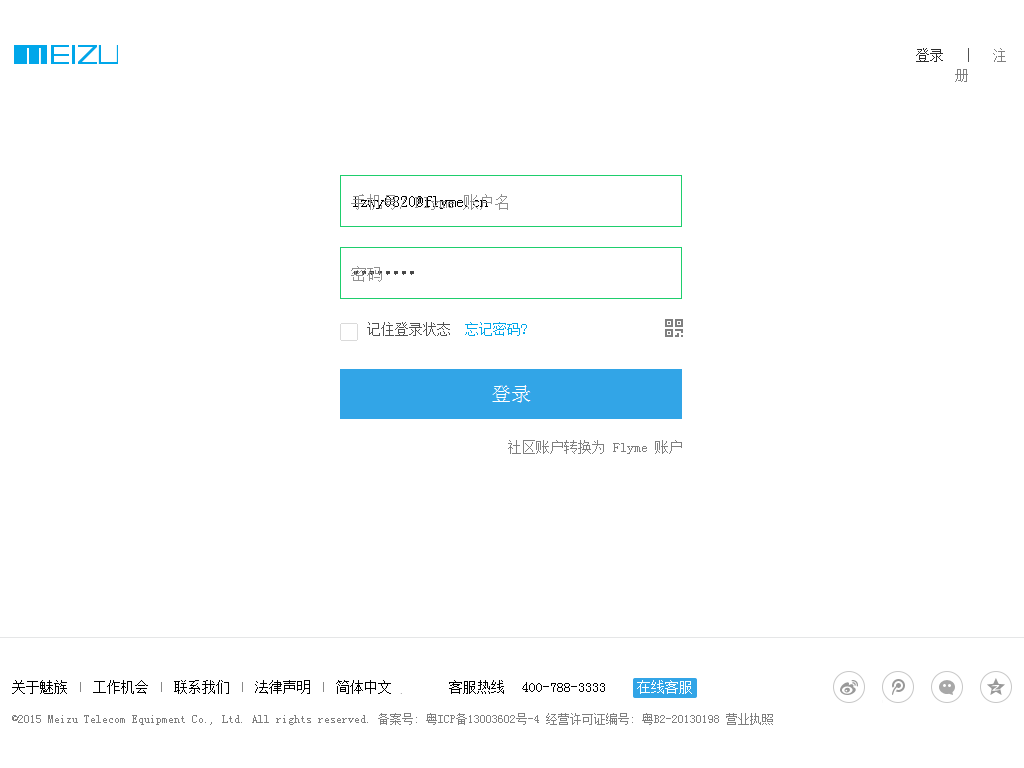
图1 登陆页
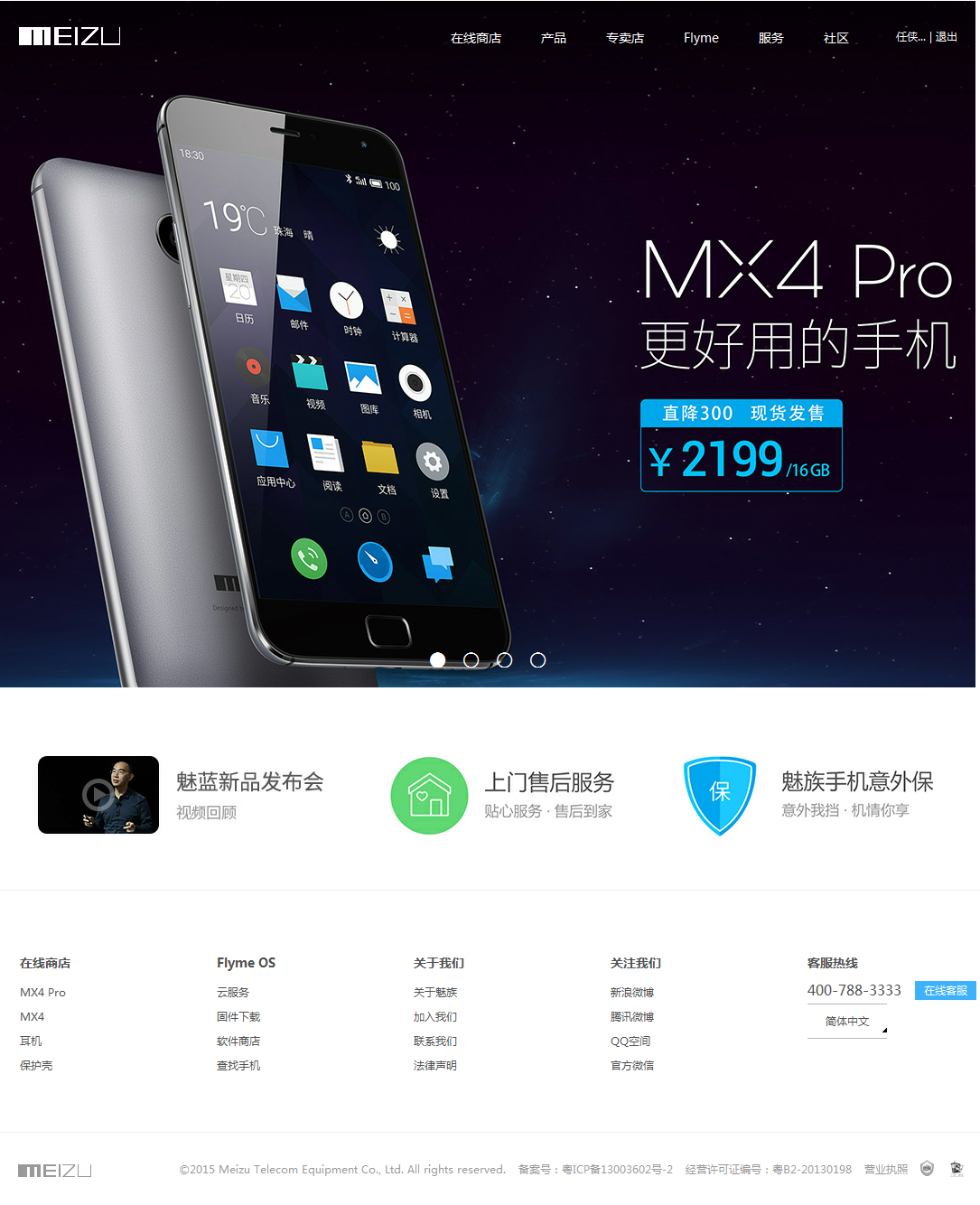
图2 首页登陆成功

图3 局部截取(header)
提示:可在 create casper 对象时进行一些初始化设置,比如不用请求图片资源,CSS资源,以及不需要的JS等资源,这样可以加快测试执行速度。
http://casperjs.org/
http://www.qiqishare.com/
三、安装与使用 SlimerJS、phantomJS 和 CasperJS
1. 安装
nodejs 安装:
http://nodejs.org
https://github.com/joyent/node/wiki/Installing-Node.js-via-package-manager
CasperJS 安装(需要 python2.6 以上版本支持):
执行命令:
npm install -g casperjs
http://docs.casperjs.org/en/latest/installation.html
https://www.python.org/download/
SlimerJS 安装(需要先安装 Firefox 或 XulRunner ):
执行命令:
npm install -g slimerjs
http://www.slimerjs.org/download.html
http://docs.slimerjs.org/current/installation.html
phantomJS 安装:
npm install -g phantomjs-prebuilt
或者下载 phantomJS:http://phantomjs.org/download.html
解压并配置 phantomjs.exe 访问路径到环境变量。
2. 使用
将上文示例保存为 js 文件(如 test_*.js) ,然后打开命令行,进入到该文件所在目录下,执行命令:
slimerjs test_slimerjs.js
phantomjs test_phantomjs.js
# 默认使用 phantomjs 引擎
casperjs test_casperjs.js
# 使用 slimerjs 引擎
casperjs test_casperjs.js –disk-cache=yes –engine=slimerjs
可对比查看执行过程与结果。
本文只是简单介绍三种工具的功能与基本安装使用,具体功能应用可参考其各自文档,也可在讨论区书写您的意见和建议。
附:前端自动化测试工具 Selenium
Selenium 也是一个前端自动化测试工具,与 casperJS 的无界面方式不同,Selenium 是直接运行于浏览器中,并且通过插件可以实现脚本录制等功能。
http://docs.seleniumhq.org/